How to Open App Store in React Native
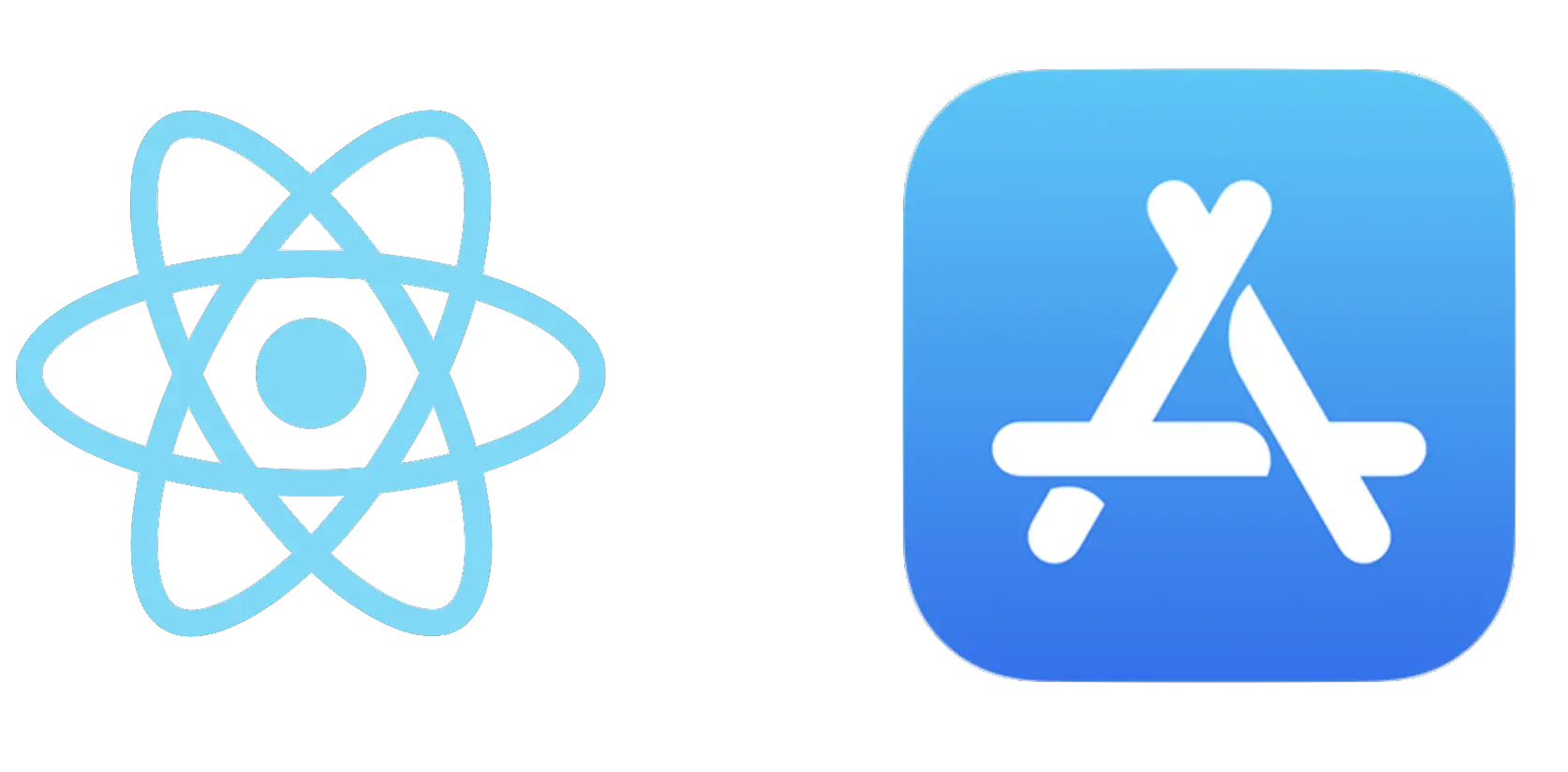
Sometimes you need to redirect App Store to download force update or something else. Its very easy to do in React Native.
For this purpose we are going to use Linking from React Native core. It means no additional package needed.
But we need to add one configuration to app Info.plist file. Its help us to open App Store directly.
<key>LSApplicationQueriesSchemes</key>
<array>
<string>itms-apps</string>
</array>
After adding this config and recompiling the app We can open App store with Linking
import React from 'react';
import {
StyleSheet,
Button,
SafeAreaView,
Linking,
} from 'react-native';
const App = () => {
const openAppStore = () => {
const link =
'itms-apps://apps.apple.com/tr/app/times-tables-lets-learn/id1055437768?l=tr';
Linking.canOpenURL(link).then(
(supported) => {
supported && Linking.openURL(link);
},
(err) => console.log(err)
);
};
return (
<SafeAreaView style={styles.container}>
<Button title="Open App Store" onPress={openAppStore} />
</SafeAreaView>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
marginHorizontal: 16,
},
});
export default App;
If you want to open Google Play Store as well check this article.
If you liked this tutorial, please subscribe and share this with your community. Cheers!