How to Open Google Play in React Native
React Native Linking to Google Play
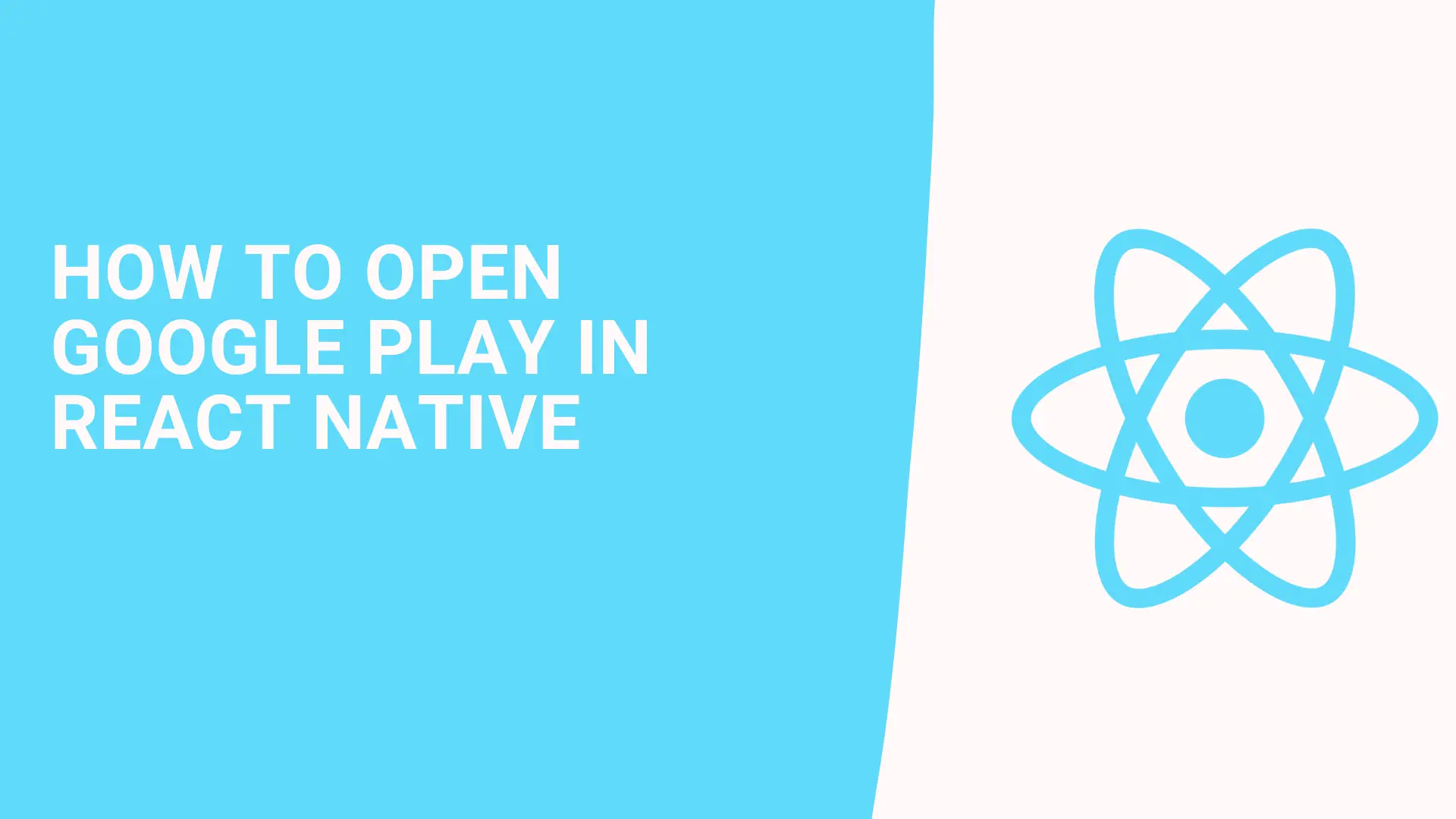
Do you need to open Google Play Store from your React Native application?
You can open it with React Native Linking API using openURL
method.
import * as React from 'react';
import { Text, View, StyleSheet, Linking } from 'react-native';
import Constants from 'expo-constants';
import { Button } from 'react-native-paper';
export default function App() {
const openGooglePlay = () => {
Linking.openURL(
'http://play.google.com/store/apps/details?id=com.google.android.apps.maps'
);
};
return (
<View style={styles.container}>
<Button mode="contained" onPress={openGooglePlay}>
Press me
</Button>
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
paddingTop: Constants.statusBarHeight,
backgroundColor: '#ecf0f1',
padding: 8,
}
});
You can try it here
Google Play provides several link formats that let you open it.
Opening Store Listing
You can use the URL format below to open deep-link directly to an app's Store listing page, where users can see the app description, screenshots, reviews and more, and then install it.
http://play.google.com/store/apps/details?id=<package_name>
Linking to a developer page
http://play.google.com/store/apps/dev?id=<developer_id>
Linking to a search result
http://play.google.com/store/search?q=<search_query>&c=apps
If you liked this tutorial, please subscribe and share this with your community. Cheers!