Optimizing React Apps: How to Cancel HTTP Requests with AbortController
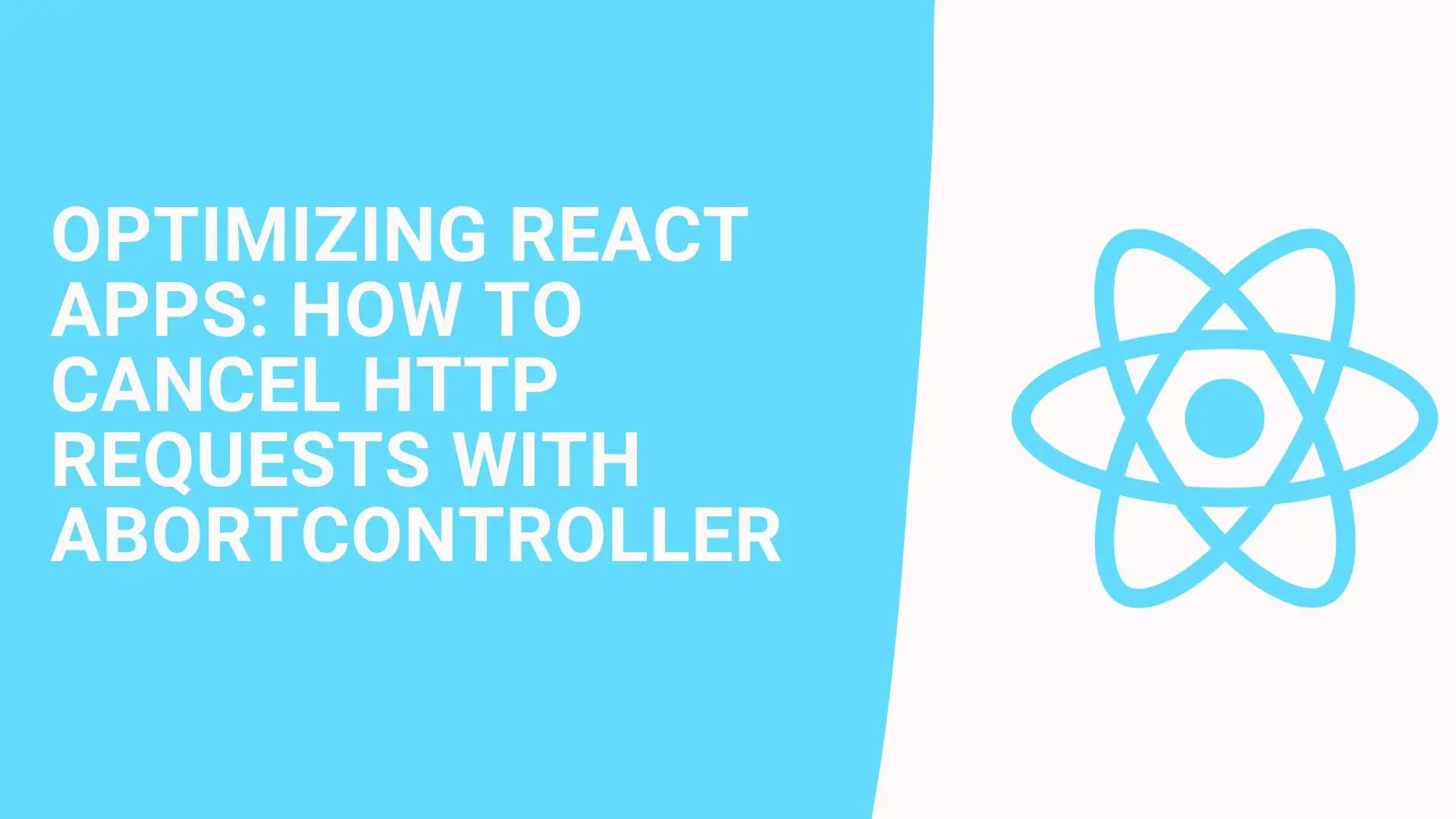
In modern web development, efficiently managing HTTP requests is crucial for enhancing application performance and user experience. React developers often encounter scenarios where ongoing HTTP requests need to be aborted—perhaps due to component unmounting or user navigating away. This is where AbortController
comes into play, offering a standardized, clean way to cancel requests. This blog post delves into how to utilize AbortController
within React applications, specifically focusing on Axios for HTTP requests.
Understanding AbortController
AbortController
is a DOM API designed for aborting one or more web requests. It provides a signal (AbortSignal
) that can be passed to fetch or Axios requests to abort them as needed. This approach is more modern and standardized compared to the older method of using cancel tokens in Axios.
Why Use AbortController?
- Standardization: As a part of the DOM API,
AbortController
is a standard method for canceling requests. - Flexibility: Works with both the Fetch API and Axios (from v0.22.0), providing flexibility across different types of HTTP requests.
- Improved Code Quality: Leads to cleaner, more maintainable code by using native JavaScript APIs.
Implementing Request Cancellation in React with Axios
Setting Up Your React Component
First, ensure your project is using Axios version 0.22.0 or later to have AbortController
support.
Step 1: Create an Instance of AbortController
Before making a request, instantiate AbortController
and use its signal in the request configuration.
import React, { useEffect, useState } from 'react';
import axios from 'axios';
const MyComponent = () => {
const [data, setData] = useState(null);
useEffect(() => {
const controller = new AbortController();
const fetchData = async () => {
try {
const response = await axios.get('https://your-api-endpoint.com/data', {
signal: controller.signal
});
setData(response.data);
} catch (error) {
if (axios.isCancel(error)) {
console.log('Request was cancelled', error.message);
} else {
console.error('An error occurred: ', error.message);
}
}
};
fetchData();
// Cleanup function
return () => {
controller.abort();
};
}, []);
return <div>{/* Render your data here */}</div>;
};
export default MyComponent;
Step 2: Cancel the Request on Component Unmount
The cleanup function in the useEffect
hook calls controller.abort()
, which cancels the request if the component unmounts.
Conclusion
AbortController
represents a modern and effective way to handle HTTP request cancellation in React applications. By leveraging this standard API, developers can ensure their applications are more performant, reliable, and maintainable. As web development practices evolve, staying updated with such best practices is crucial for building high-quality React apps.