How to Retrieve the Build and Version Number of Your React Native App
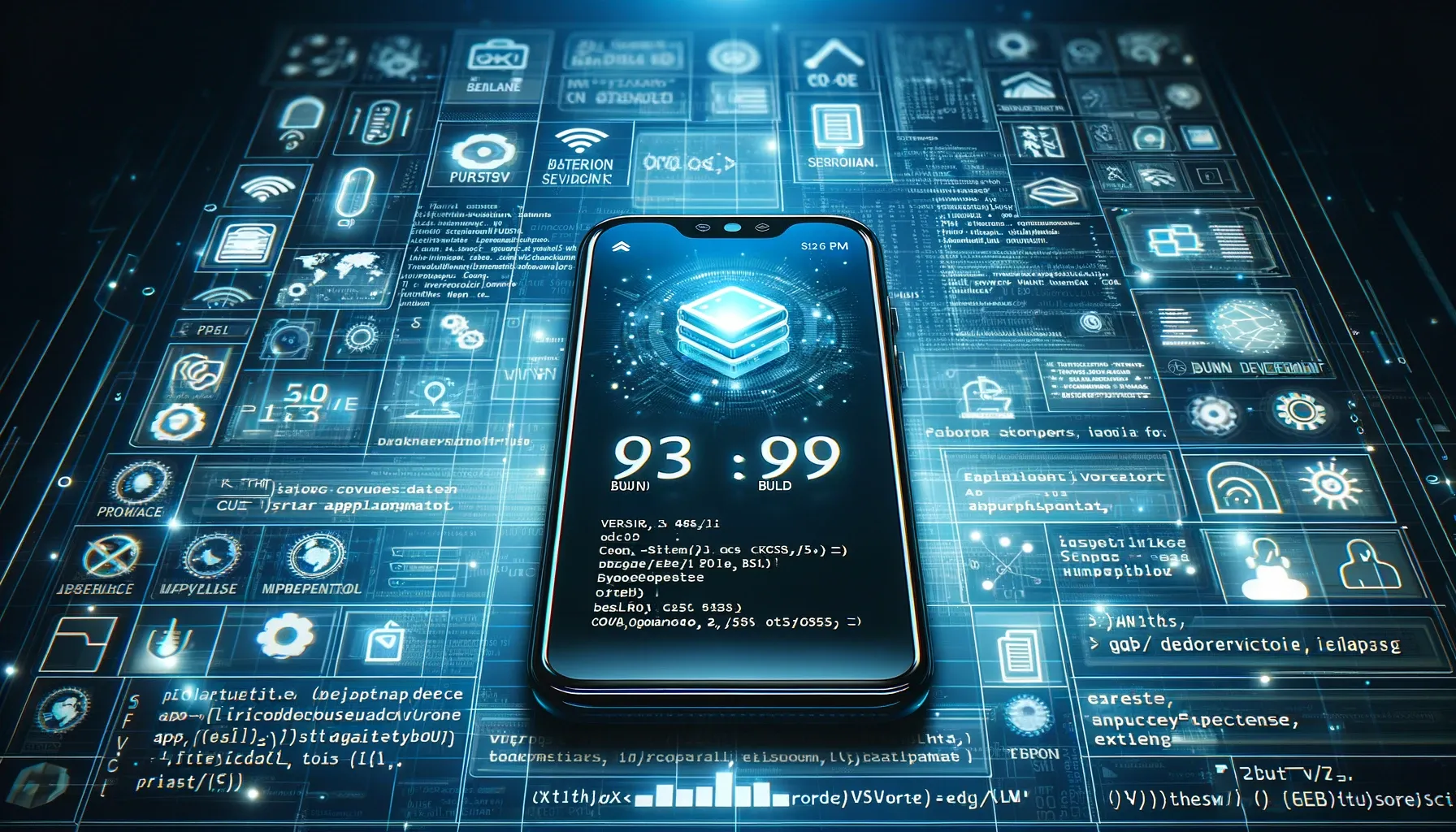
In the world of mobile app development, keeping track of different versions and builds of your application is essential for managing releases, debugging, and providing user support. For React Native developers, one efficient way to access these crucial pieces of information is by utilizing the react-native-device-info
library. This powerful tool not only grants access to your app's version and build number but also offers a plethora of device-related data, enhancing your ability to fine-tune your app's performance and user experience.
Step 1: Installing react-native-device-info
The first step in harnessing the power of react-native-device-info
is to add it to your project. This process is straightforward and can be accomplished with a simple command. Open up a terminal, navigate to your project directory, and execute one of the following commands based on your package manager preference:
Using Yarn:
yarn add react-native-device-info
Using NPM:
npm install --save react-native-device-info
Step 2: Linking the Library (For Older React Native Versions)
If your project is based on React Native version below 0.60, you'll need to manually link the library to your project. This is a necessary step to ensure that the native code bundled with the library is correctly integrated with your app's build process. Run the following command to achieve this:
react-native link react-native-device-info
For projects using React Native 0.60 and above, the auto-linking feature simplifies this process by automatically handling the linking for you.
Step 3: Accessing Version and Build Number
With react-native-device-info
successfully integrated into your project, you can now easily access the app's version and build number within your JavaScript code. Import the library and use its methods to retrieve the information you need:
import DeviceInfo from 'react-native-device-info';
// Get the app version
const appVersion = DeviceInfo.getVersion();
// Get the build number
const buildNumber = DeviceInfo.getBuildNumber();
Step 4: Utilizing the Version and Build Number
After obtaining the version and build number, you're ready to incorporate this data into your app's functionality. Whether you're displaying it within the UI for reference, logging it for debugging purposes, or using it to manage user support queries, integrating this information is simple and straightforward:
import React from 'react';
import { View, Text } from 'react-native';
const AppInfo = () => (
<View>
<Text>App Version: {DeviceInfo.getVersion()}</Text>
<Text>Build Number: {DeviceInfo.getBuildNumber()}</Text>
</View>
);
export default AppInfo;
This basic example showcases how to display the app version and build number, but the potential uses extend far beyond this. Tailor the implementation to fit your specific needs, ensuring that your app and its development process benefit fully from this valuable data.
Conclusion
Leveraging react-native-device-info
to access your React Native app's build and version number is a testament to the flexibility and power of the React Native ecosystem. Beyond these capabilities, the library offers extensive device and system information that can significantly enhance your development and debugging workflows. As always, remember to thoroughly test any new integration across different platforms and devices to ensure consistent behavior and performance for your users.
Stay tuned for more insights and tips on making the most of your React Native projects!