Anatomy of AndroidManifest.xml: Understanding the Backbone of Android Apps
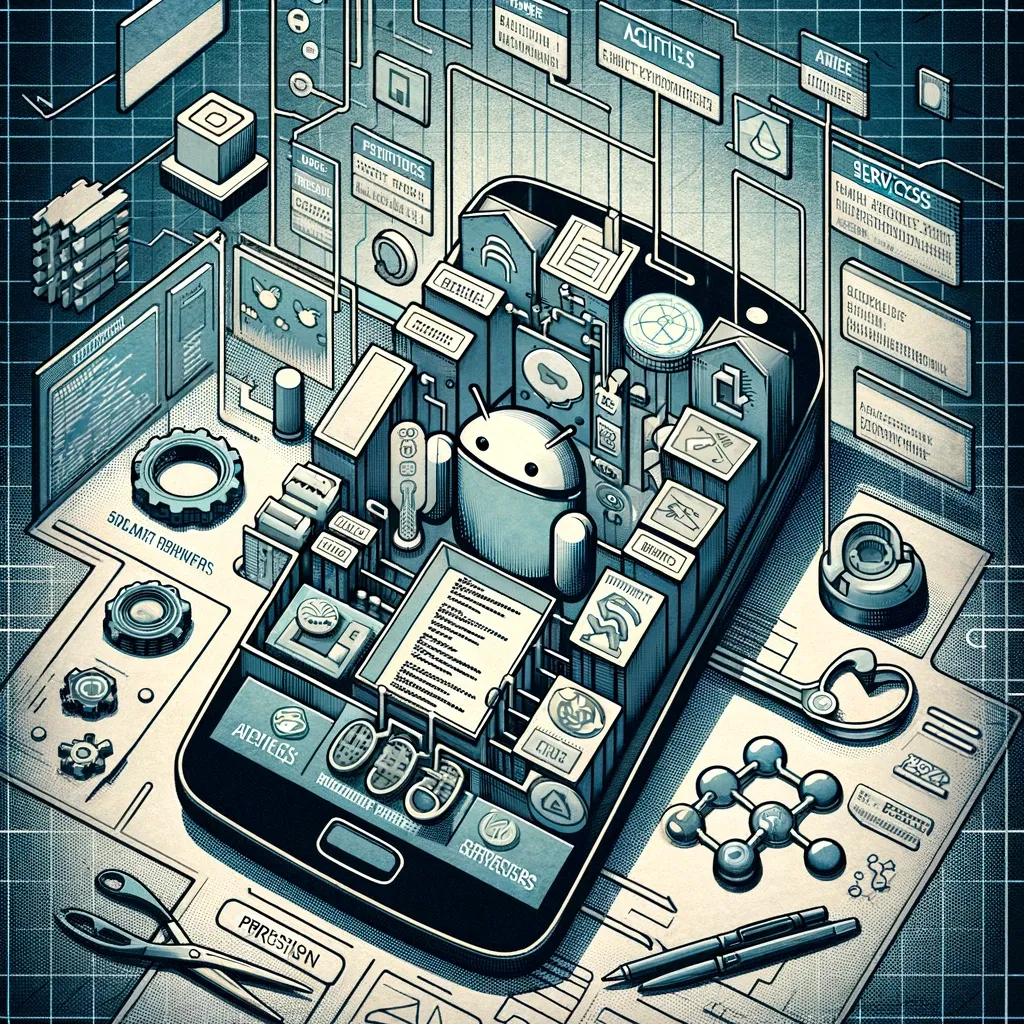
AndroidManifest.xml is the cornerstone of any Android application. This file, nested at the root of the app project, acts as a central hub for essential information about the application. It serves several critical functions, including defining the app's package name, components (activities, services, broadcast receivers, and content providers), required permissions, and hardware and software features. Understanding the anatomy of the AndroidManifest.xml file is crucial for both new and experienced Android developers. Let's dive into the key elements that make up this vital file.
1. The Package Name
At the very beginning, the manifest
element declares the package name of the application. This name is unique to the app and is used by the system to identify it among other apps on a device. It's also used as a namespace for the app's components.
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.myapp">
2. Application Components
AndroidManifest.xml defines four types of application components:
- Activities (
<activity>
): These are the entry points for user interaction. Each activity represents a single screen with a user interface. - Services (
<service>
): These run in the background to perform long-running operations or to work for remote processes. - Broadcast Receivers (
<receiver>
): They listen for and react to broadcast messages from other applications or from the system itself. - Content Providers (
<provider>
): These manage a shared set of application data that you can store in the file system, in an SQLite database, on the web, or on any other persistent storage location that your app can access.
3. Permissions
Permissions play a critical role in Android security by protecting the privacy of users. The uses-permission
tag is used to request the necessary permissions for the app. For example:
<uses-permission android:name="android.permission.CAMERA" />
Permissions ensure that sensitive information is only accessed by apps with explicit user permission.
4. Hardware and Software Features
The uses-feature
element declares the hardware and software features the app requires, such as a camera or internet access. This helps ensure that apps are run on compatible devices.
<uses-feature android:name="android.hardware.camera" android:required="true" />
5. Application Node
The application
node provides essential information about the app, including the icon, theme, and any global data. It's also where you declare the components and their properties.
<application
android:icon="@drawable/ic_launcher"
android:label="@string/app_name">
<!-- Declare components like activities here -->
</application>
6. Intent Filters
Intent filters within an activity, service, or receiver components declare the types of intents that the component is willing to accept. This mechanism is how activities are launched and how services and receivers can be triggered by other parts of the system or different apps.
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
7. Versioning
Versioning information is critical for Android applications. The android:versionCode
and android:versionName
attributes within the manifest
tag declare the version of the app, which is important for app updates through the Google Play Store.
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.myapp"
android:versionCode="1"
android:versionName="1.0">
Conclusion
The AndroidManifest.xml file is the skeleton of an Android application. It outlines the structure, defines the app's components, declares permissions, and specifies hardware and software features. Understanding its elements and how they work together is essential for developing functional, secure, and efficient Android applications. Whether you're a beginner learning the ropes or a seasoned developer, revisiting the anatomy of AndroidManifest.xml can provide valuable insights into the best practices of Android app development.