How to use React Hook Form in React Native
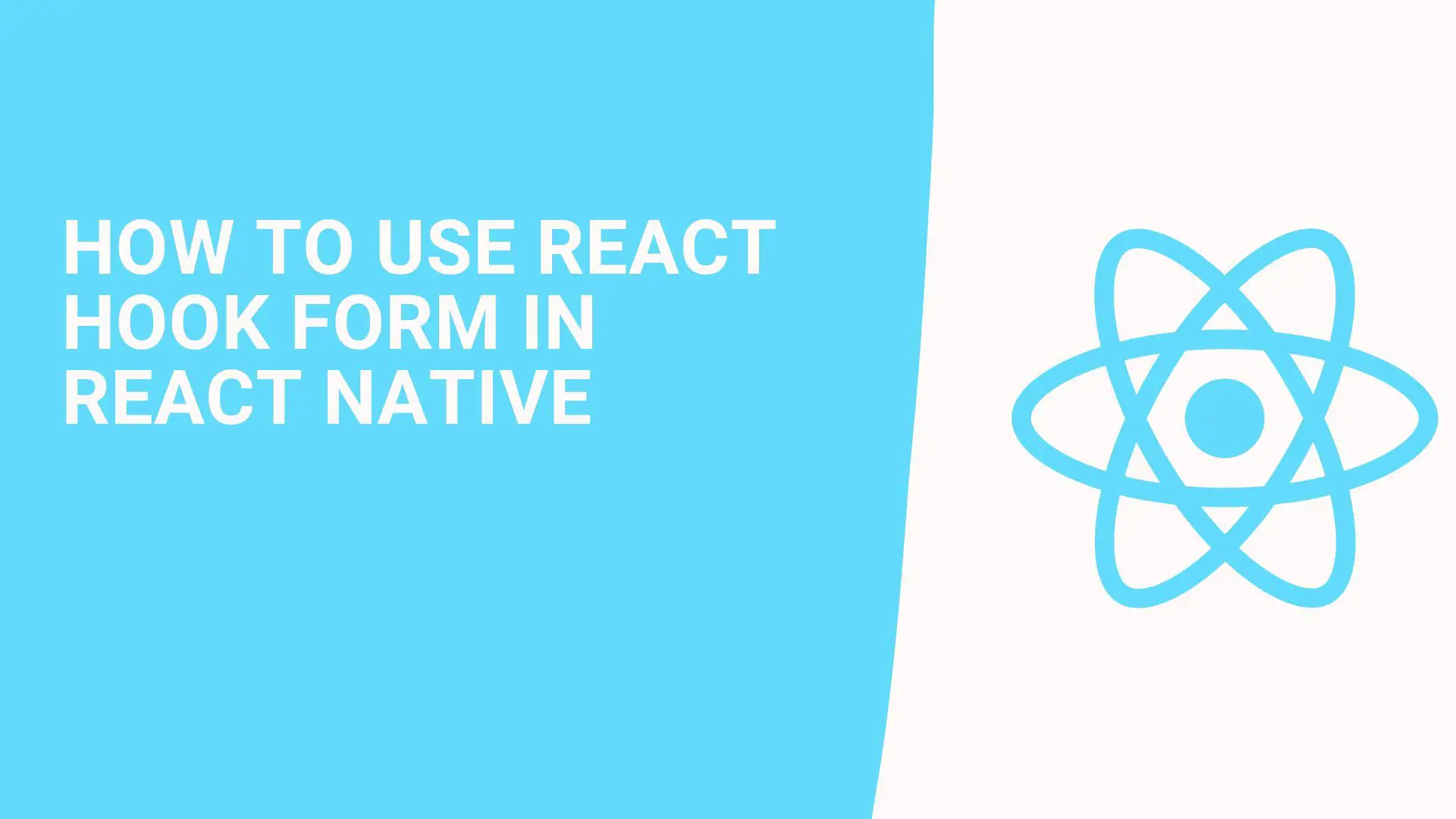
Using React Hook Form in React Native is a great way to manage forms efficiently, providing a seamless way to handle user input and validation. React Hook Form is a library that helps you validate forms in React and React Native applications. It reduces the amount of boilerplate code you need to write and improves performance by minimizing re-renders.
Here's a step-by-step guide on how to use React Hook Form in a React Native project:
1. Installation
First, you need to install React Hook Form in your project. You can do this using npm or yarn:
npm install react-hook-form
or
yarn add react-hook-form
2. Importing React Hook Form
In your component file, import the useForm
hook from React Hook Form:
import { useForm, Controller } from 'react-hook-form';
3. Initializing the Form
Use the useForm
hook to initialize the form. It returns an object with methods that you can use to manage your form, such as handleSubmit
, control
, and formState
.
const { control, handleSubmit, formState: { errors } } = useForm();
4. Creating the Form
Use the Controller
component from React Hook Form to wrap your input components. The Controller
makes it easy to integrate with React Native components like TextInput
.
Here's a basic example of a form with a single TextInput
for an email field:
import React from 'react';
import { View, TextInput, Button, Text } from 'react-native';
import { useForm, Controller } from 'react-hook-form';
const MyForm = () => {
const { control, handleSubmit, formState: { errors } } = useForm();
const onSubmit = data => console.log(data);
return (
<View>
<Controller
control={control}
rules={{ required: true }}
render={({ field: { onChange, onBlur, value } }) => (
<TextInput
onBlur={onBlur}
onChangeText={onChange}
value={value}
style={{ borderColor: 'gray', borderWidth: 1, marginBottom: 20 }}
/>
)}
name="email"
/>
{errors.email && <Text>This is required.</Text>}
<Button title="Submit" onPress={handleSubmit(onSubmit)} />
</View>
);
};
export default MyForm;
5. Handling Submissions
The handleSubmit
method from the useForm
hook is used to handle form submissions. Pass a callback function to handleSubmit
that will be executed when the form is valid.
const onSubmit = data => console.log(data);
In the example above, the onSubmit
function will log the form data to the console.
6. Validation
You can add validation rules directly to the Controller
component using the rules
prop. React Hook Form supports a wide range of validation strategies, including required fields, pattern matching, and custom validation functions.
7. Displaying Error Messages
The formState
object contains information about the form's state, including any errors. You can use this to display error messages in your UI.
{errors.email && <Text>This is required.</Text>}
In the example above, an error message is displayed if the email
field is empty.
Conclusion
React Hook Form simplifies form handling in React Native applications, making it easier to manage form state, perform validation, and handle submissions. By following these steps, you can efficiently integrate React Hook Form into your React Native project.