How to Integrate Maps in React Native
Maps are an important part of the apps. In this blog post, we are going to integrate the React Native Maps package into our sample app.
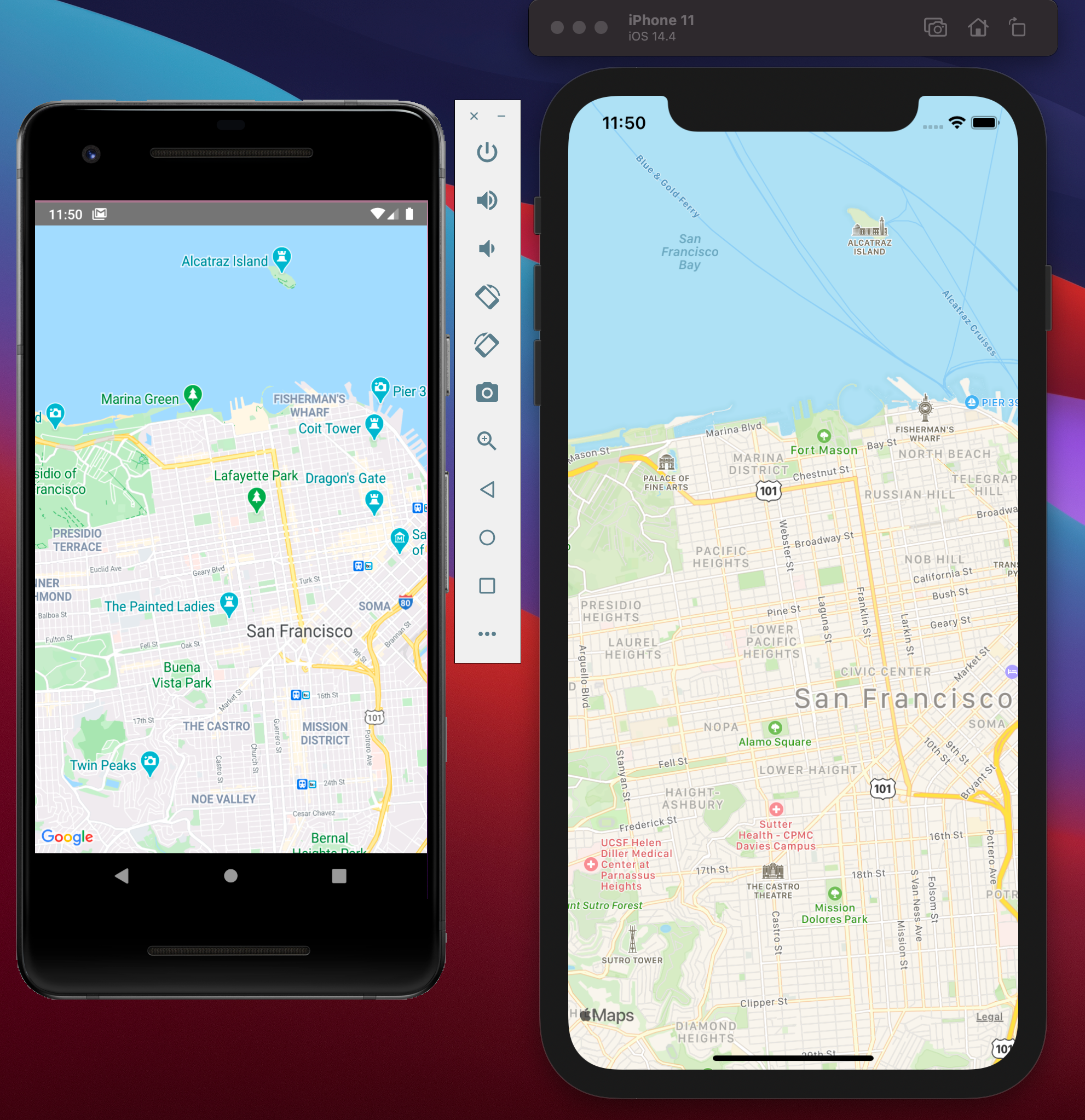
Maps are an important part of the apps. In this blog post, we are going to integrate the React Native Maps package into our sample app.
So, let’s get started
1- Create an app if you don't have one yet.
npx react-native init MapsExample
2- Add React Native Maps package.
yarn add react-native-maps -E
3 - For iOS we have to install Cocoapods modules.
npx pod-install or cd ios && pod install
4- For Android we are using Google Maps, So we have to configure Google Maps API key. You can find this file inside android/app/src/main/AndroidManifest.xml.
<application>
<!-- You will only need to add this meta-data tag, but make sure it's a child of application -->
<meta-data
android:name="com.google.android.geo.API_KEY"
android:value="Your Google maps API Key Here"/>
<!-- You will also only need to add this uses-library tag -->
<uses-library android:name="org.apache.http.legacy" android:required="false"/>
</application>
Due to the nature of React Native. when you add or change native code to the app. we need to recompile for both platforms.
Now we are ready to test Map in our app. just copy and paste this code inside your App.js (if you have empty app)
import React from 'react';
import MapView from 'react-native-maps';
import {StyleSheet, View} from 'react-native';
const App = () => {
return (
<View style={{flex: 1}}>
<MapView
style={styles.map}
initialRegion={{
latitude: 37.78825,
longitude: -122.4324,
latitudeDelta: 0.0922,
longitudeDelta: 0.0421,
}}
/>
</View>
);
};
const styles = StyleSheet.create({
map: {
...StyleSheet.absoluteFillObject,
},
});
export default App;
If you liked this tutorial, please subscribe and share this with your community. Cheers!