Android Development with Kotlin — Linear Layout
In this tutorial, we are going to learn how to create Linear Layout in our applications.
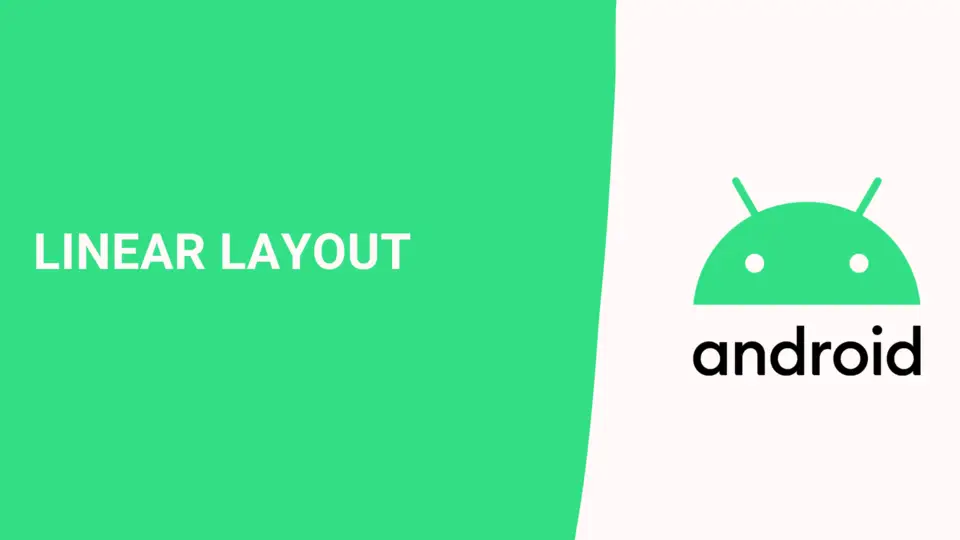
What is Linear Layout?
Linear layout is a simple layout used in Android for layout designing. In the Linear layout, all the children's elements are displayed according to their orientation. Orientation attribute can be either horizontal
or vertical
.
Let’s look at some code to understand the attributes that help us to configure a linear layout and its child controls.
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="wrap_content">
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Button1"
android:id="@+id/button"
android:backgroundTint="#358a32" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Button2"
android:id="@+id/button2"
android:backgroundTint="#0058b6" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Button3"
android:id="@+id/button3"
android:backgroundTint="#c62828" />
</LinearLayout>
In the above, we didn't specify any orientation because by default LinearLayout horizontal
orientation.
if you check the result, buttons are shown as stacked to each other.
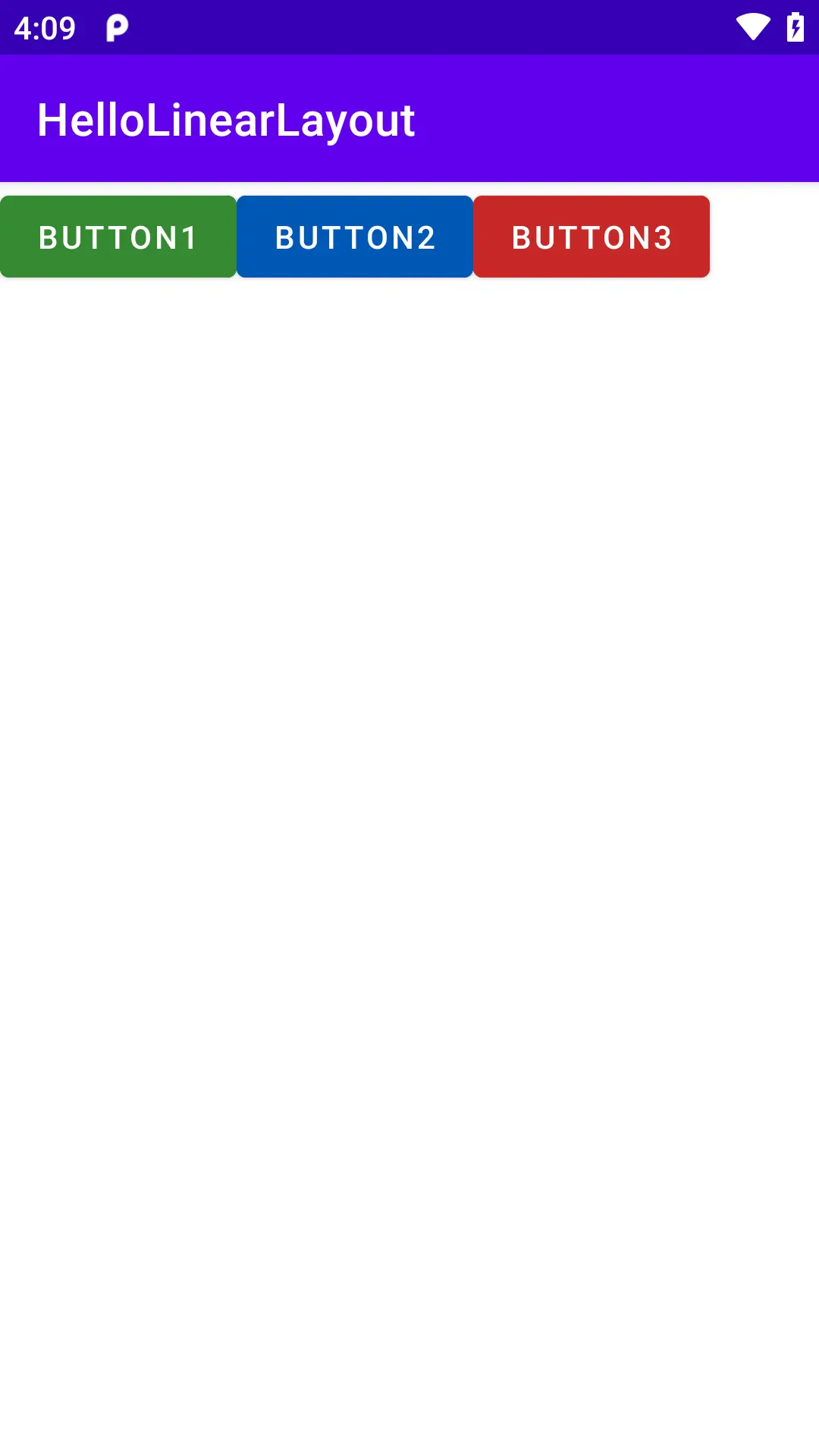
if we specify the orientation of LinearLayout which is vertical
, all the elements will be stacked vertically.
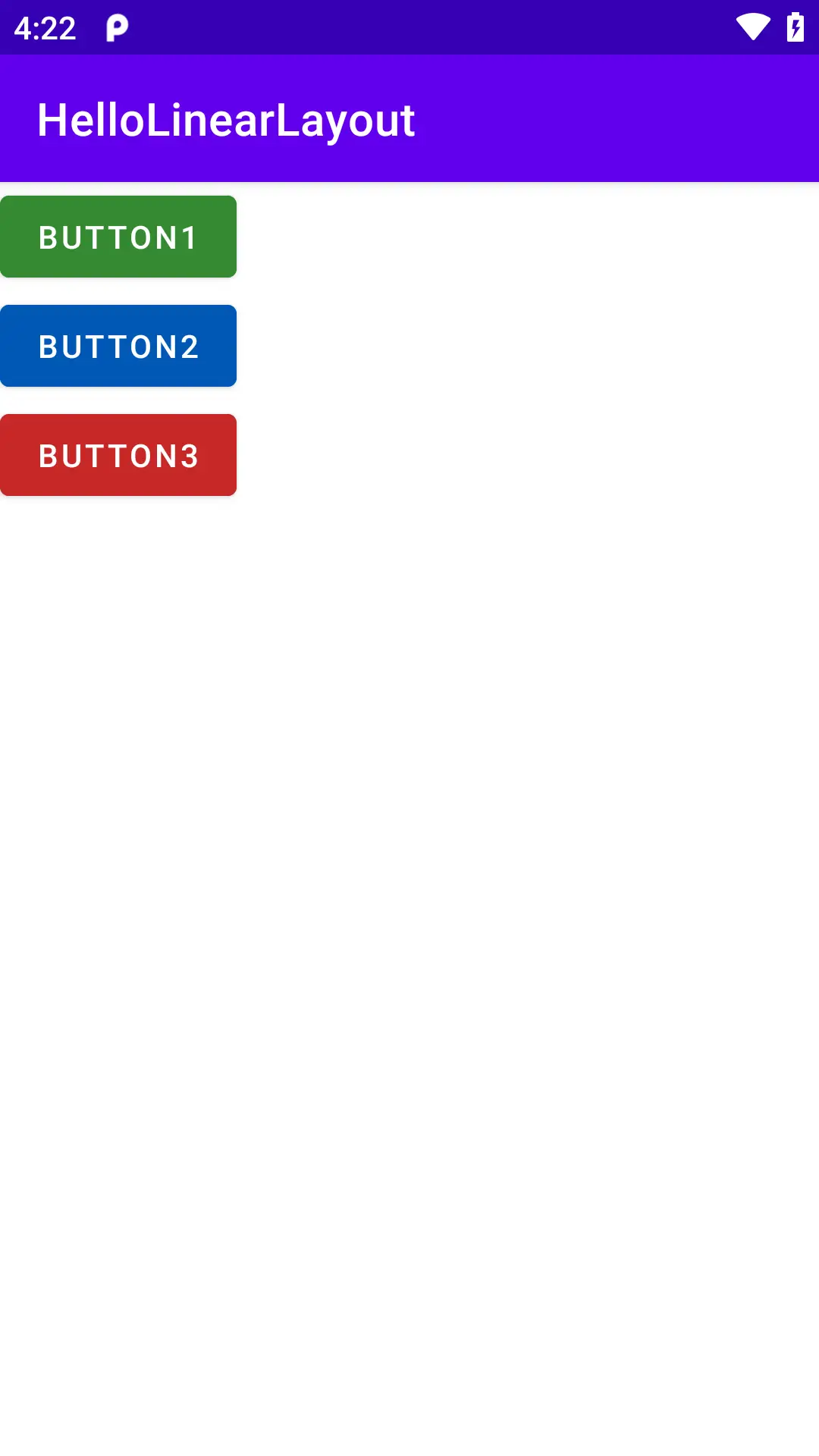
The second most important attribute is layout_weight
which is used to distribute whatever left space into proportions. Default layout_weight
is zero.
Let’s look at some code to understand briefly
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:background="@color/teal_200"
android:orientation="horizontal">
<Button
android:id="@+id/button"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:backgroundTint="#358a32"
android:text="Button1" />
<Button
android:id="@+id/button2"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:backgroundTint="#0058b6"
android:text="Button2" />
<Button
android:id="@+id/button3"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:backgroundTint="#c62828"
android:text="Button3" />
</LinearLayout>
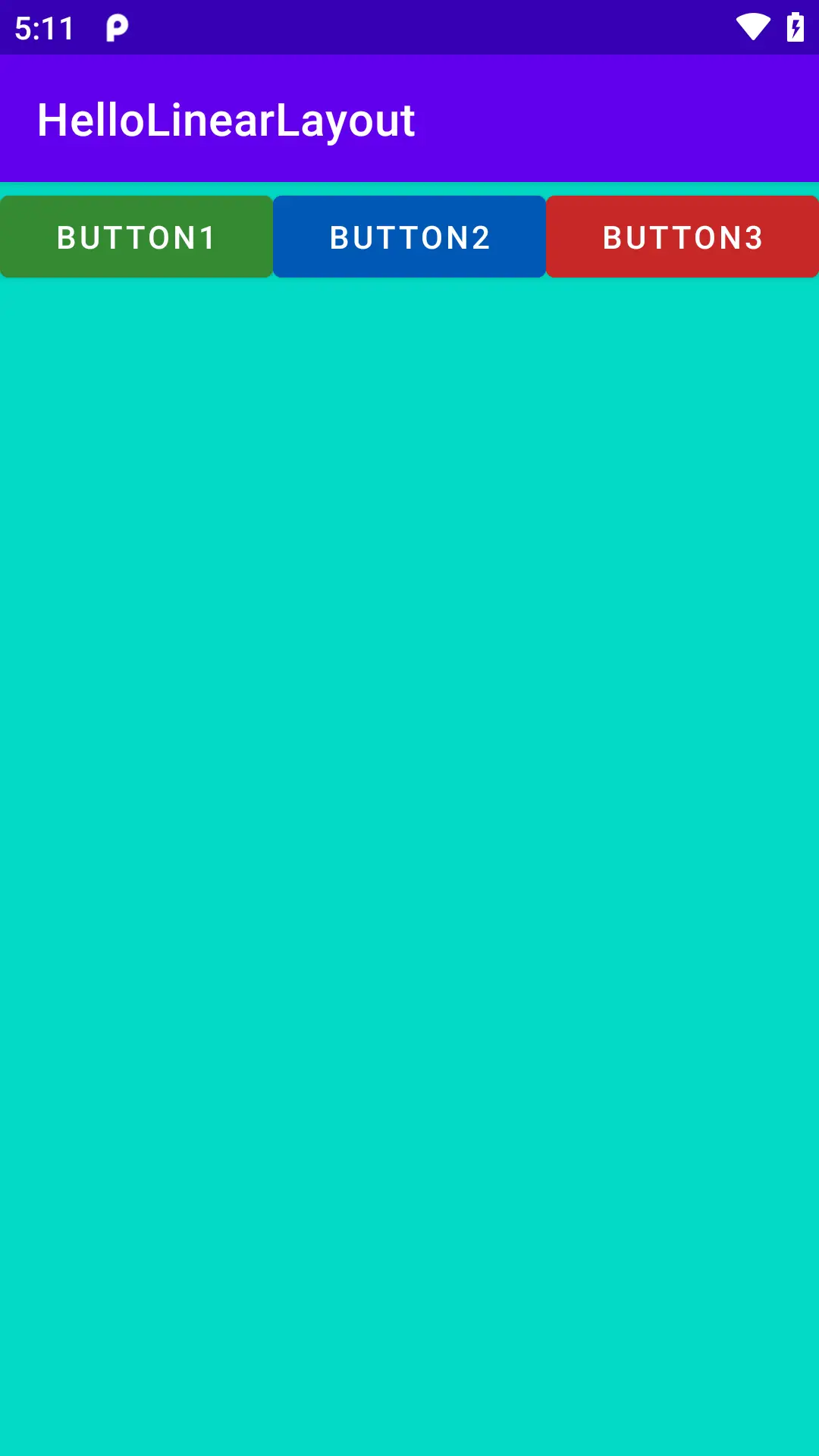
If you check the above code and screenshot we added the layout_weight="1"
attribute for each children element to distribute equally.
You may also notice we also changed layout_width="0dp"
. It means layout_width
attribute will be ignored.